Margin and translation
Most chart types use a bottom and a left axis.
In these cases, we need to leave space for tick labels and axis titles. Let’s look at how to implement this effectively.
SVG Area and Bounds Area
Imagine an SVG area with a width
of 500px and a height
of 300px.
The left and bottom axes aren’t displayed right at the SVG border. Instead, we add margins on all sides: left, right, bottom, and top.
The area within these margins is known as the bounds, where the chart content is positioned between the x and y axes. In our code, we’ll refer to the width and height of this bounds area as boundsWidth
and boundsHeight
.
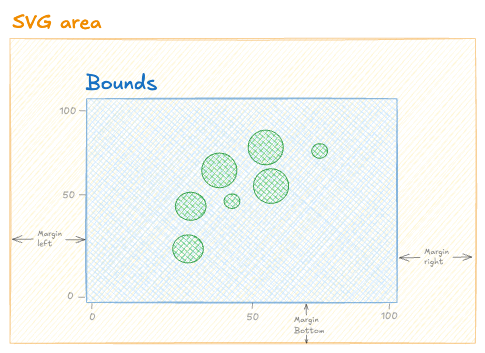
Anatomy of the chart areas: some margins are set all around the SVG area. The area inside is called the Bounds.
Implementation
A chart component often starts by defining its margins. An object with 4 properties is ideal for that:
const MARGIN = {
top: 10,
right: 30,
bottom: 50,
left: 30
}
Since the chart component has defined width
and height
, we can easily determine the dimensions of the bounds area, referred to as boundsWidth
and boundsHeight
:
const boundsWidth = width - MARGIN.right - MARGIN.left;
const boundsHeight = height - MARGIN.top - MARGIN.bottom;
🪄 The tricky part
Finally the svg is going to be rendered like this:
<svg width={width} height={height}>
<g
width={boundsWidth}
height={boundsHeight}
transform={"translate(" + MARGIN.left + " , " + MARGIN.top].join(',')})"}
>
// ... all shapes go here
</g>
</svg>
What's going on here? 😱
1️⃣ The SVG area is created as usual with the svg
element, along with the specified width
and height
.
2️⃣ The g
element is used to group other SVG elements, similar to how a div
works in HTML. This group represents the bounds, defined by its boundsWidth
and boundsHeight
dimensions!
3️⃣ The transform
property is used to translate the bounds slightly to the right and down, creating space for the left and top margins!
Exercices
Drawing the Axis
Now that we’ve created space for it, it’s time to draw the axis. Let’s build some reusable components for this!