Other scale types
While scaleLinear
is one of the most commonly used D3 scales, other scale types are essential for creating even basic charts.
Let's explore scaleBand
, scaleOrdinal
, and other indispensable functions!
scaleBand()
to create... bands π
scaleBand()
is ideal for categorical variables as it allocates equal space for each category, ensuring that every discrete value is uniformly represented on the axis.
Example
You want to create a horizontal bar chart with 3 bars in a figure that is 240 pixels high. You want to dedicate 33% of the total height for white space: this is the padding between bars.
You'll end up with the dimensions outlined below:
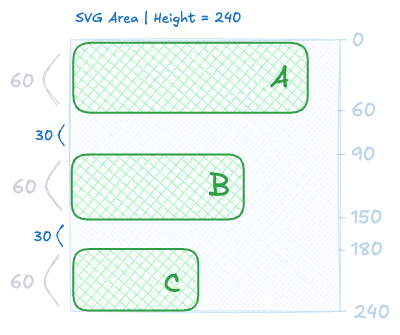
All the numbers produced by the scaleBand()
function.
You can easily compute these values using the scaleBand()
function!
There are many ways to control the padding
. The official doc explains very well!
- The
domain
is an array that lists the groups. - The
range
is a two-element array that specifies the pixel positions where the shapes will be drawn. - The optional
padding
method is expressed as a fraction of the band width.
const yScale = d3.scaleBand()
.domain(['A', 'B', 'C'])
.range([0, height])
.padding(0.1)
Now that this scale is available, let's use it to get all the positions we need! Note that the yScale
function we now have returns the start
of the corresponding band:
// Top of each bar:
console.log(yScale('A')); // 0
console.log(yScale('B')); // 90
console.log(yScale('C')); // 180
// Width of a bar:
console.log(yScale.bandwidth()); // 60
That's it! We have all the numbers we need to make a barplot! π
TODO: add a simulator to make ppl understandpadding
,innerPadding
andouterPadding
.
Scales are not just for positioning: scaleOrdinal()
Scales are a fundamental concept in data visualization, and they are not limited to positioning elements!
scaleOrdinal()
is a great example: it maps a set of discrete values to another set of discrete values. For instance, it can be used to assign a specific color to each group name.
const colorScale = d3.scaleOrdinal()
.domain(["a", "b", "c"]) // I have 3 groups in the dataset: a, b, c
.range(["red", "green", "blue"]) // I want to assign 3 colors to them
colorScale("a") // --> red!
colorScale("b") // --> green
More Scale Types to Explore!
The d3-scale
module includes 13 different types of scales.
However, they all follow the same core pattern. Rather than covering each one individually now, we'll explore them throughout the course as they come up.
By now, you should have a solid understanding of how scales work.
While I donβt recommend it, you can always check out the full list of scale types in the official documentation if you're curious!
Exercices
It's taking shape! π
You've now mastered two fundamental concepts of dataviz with React and D3: SVG and Scales. This means we're ready to build actual graphs!
In the next lesson, you'll dive into a hands-on exercise where we recreate a real-world chart using everything you've learned so far.
Let's do it! π