Building shapes with d3
Some shapes can be quite complex—like rings, slices, paths, polygons, and curves. Manually creating the d
attribute for these shapes would be a nightmare!
Fortunately, D3 provides a module called d3-shape
specifically for handling all these shapes. Let’s explore how it can simplify our work.
Building the line you need
In the previous lesson we introduced the path
element, and showed that a line chart is basically rendered this way:
<svg height="300" width="400">
<path
d="M0 105 L100 200 L200 200 L300 20 L350 120"
fill="none"
stroke="#69b3a2"
/>
</svg>
We also learned that d3 includes a module called d3-shape
, which offers a variety of helper functions for building shapes. These functions take your data and automatically generate the d
attribute for a path!
Now, let’s explore how to use the d3.line()
function to create the path for a line chart:
const data = [
{ x: 0, y: 40 },
{ x: 50, y: 70 },
{ x: 100, y: 150 },
...
];
Everything begins with a data
object, which is almost always an array.
Each item in the array represents a data point and is usually an object with properties.
For a line chart like the one above, you'll need at least two properties: one for the x-axis position and one for the y-axis position.
Money back! The syntax is weird!
When I first started using d3, I found this method chaining syntax very frustrating / weird / unusual / hard to read.
I was more used to functions that take parameters and do stuff with it:
// Create a function that expects 2 parameters
const multiply = (x, y) => x * y;
// Use the function, providing it the 2 parameters
multiply(4, 6)
// 24
But that's how d3.js works, and I promise you will soon be used to it!
Other helpers
The d3.line()
function is one of the most versatile in d3, enabling the creation of key chart types like line charts, lollipop plots, and even radar charts.
However, you can’t use it to create a donut chart! For that, you’ll need to draw arcs, and d3 provides a specific helper for this: d3.arc()
.
The d3-shape
module includes 15 functions in total. Here's a diagram that highlights the most important ones, which can help you build about 80% of chart types!
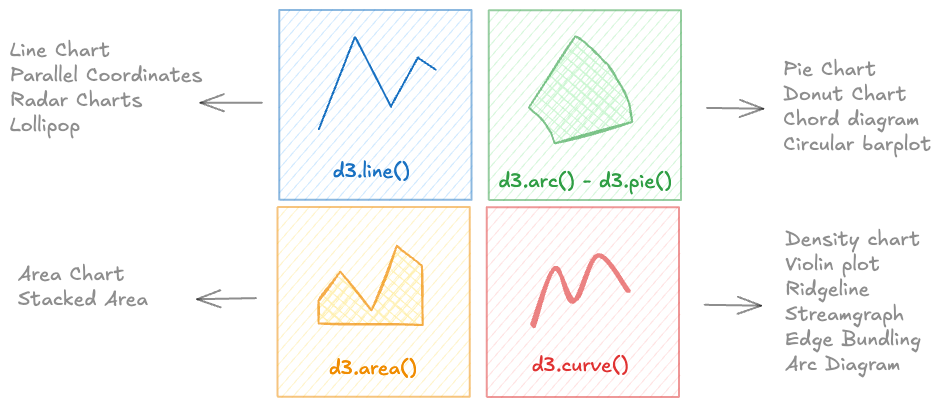
Main functions of the d3-shape
module
Describing each of these functions in detail one by one would be tedious. Instead, we’ll introduce them gradually throughout the course.
What’s crucial to remember is that they all share a common structure: path generators, method chaining, and accessor functions are key concepts you'll need to fully grasp.